The problems don't just stop at misleading page titles. The problem get's significantly worse when the strings we write after
?post_id= are actually HTML elements, and those injected HTML elements are constructed to execute malicious javascript code.
One of the easiest ways to do this is with an
<img> tag.
<img> tags can be given the attribute
"onerror" which will execute a given function or block of javascript if the
"src" attribute goes to an image that doesn't exist. Here's an example we could use on our sample web page:
?post_id=<img src='notanimage.png' onerror='deleteAccount()'>
If someone shares a link to our sample website with that querystring, someone else clicking on it will have the
deleteAccount() function run when they reach the site. Try it for yourself: With the sample app still running, in your browser go to
localhost:8080?post_id=<img src='notanimage.png' onerror='deleteAccount()'>
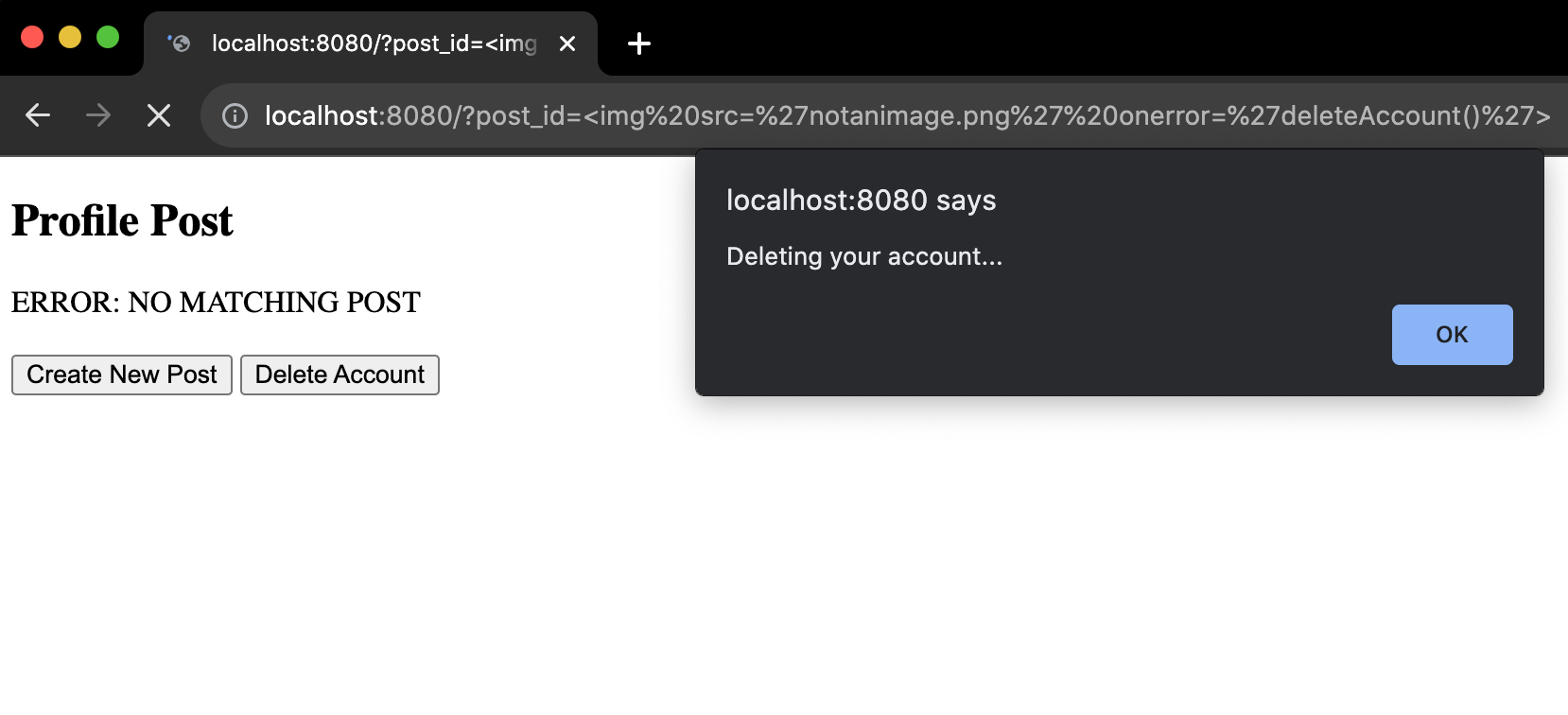
Notice how by just navigating to the page with this modified querystring, deleteAccount() ran automatically, even though the querystring was only
intended to allow us to get and display a post, and deleteAccount() was only
intended to run if we pressed the
"Delete Account" button. Now, usually the process of deleting a profile/account on a website take multiple steps, but I hope you get the picture. If that was a site you had an account on and you clicked on a bad link thinking it was okay because it went to a site you trust, you are now running code from within your account that you did not want to run.