Reverse Engineering the Second Crackme:
Extract the second crackme to your crackmes directory (same as in Part 1) using the password “crackmes.one”, if you haven’t done so already. This should add a Unix executable to that directory with the name “crack1_by_D4RK_FL0W”. Open up terminal, cd into your crackmes directory and go ahead and run the executable:
$ ./crack1_by_D4RK_FL0W
Now enter “test” to see what to expect next. You should see something like this:
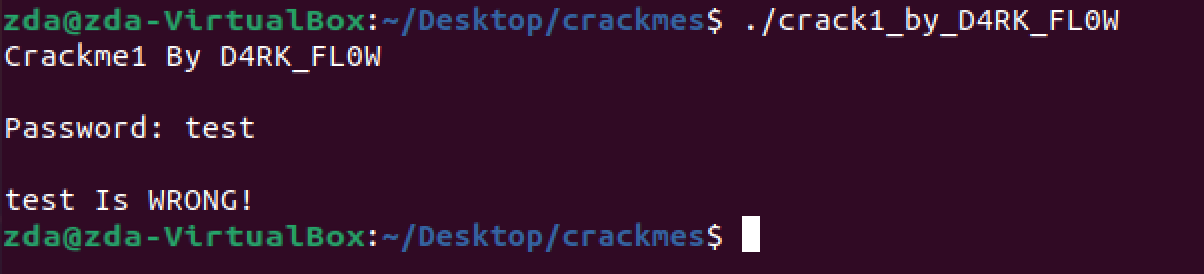
Okay, looks like we have another basic “figure out the password” crackme. Once again let’s start with
strings:
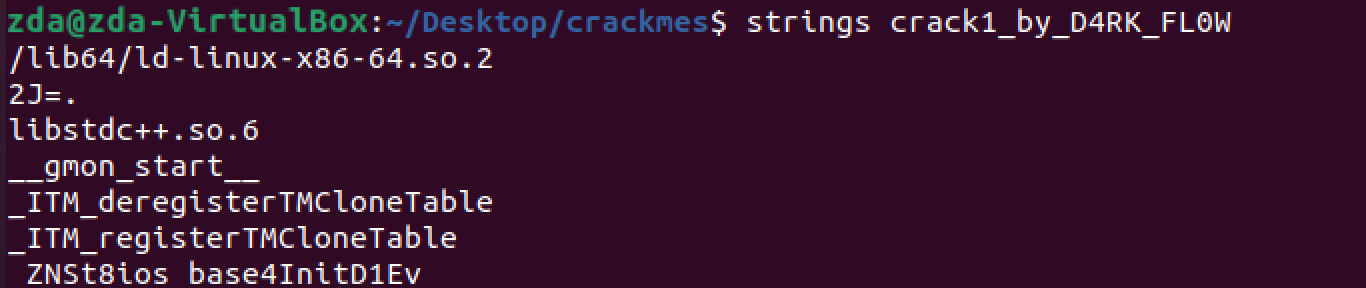
If you scroll through the output you can see on this list some of the text that we saw when we ran the program:
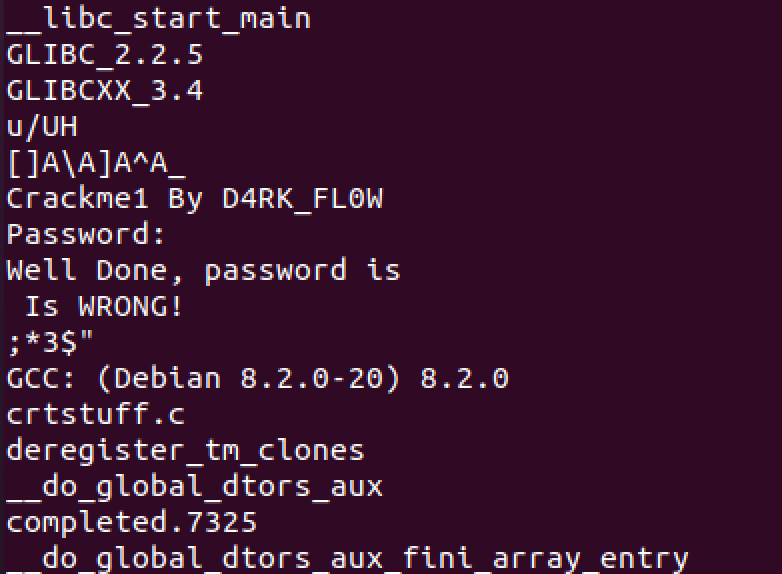
It also looks like this program was written on Debian 8.2.0 and compiled with gcc. Unfortunately nothing stands out as an obvious password, so we’ll try something else. In Part 1, we had success using
ltrace, a dynamic analysis tool. Let’s see if we have any luck here:
$ ./ltrace ./crack1_by_D4RK_FL0W
And enter “test” again for the password:
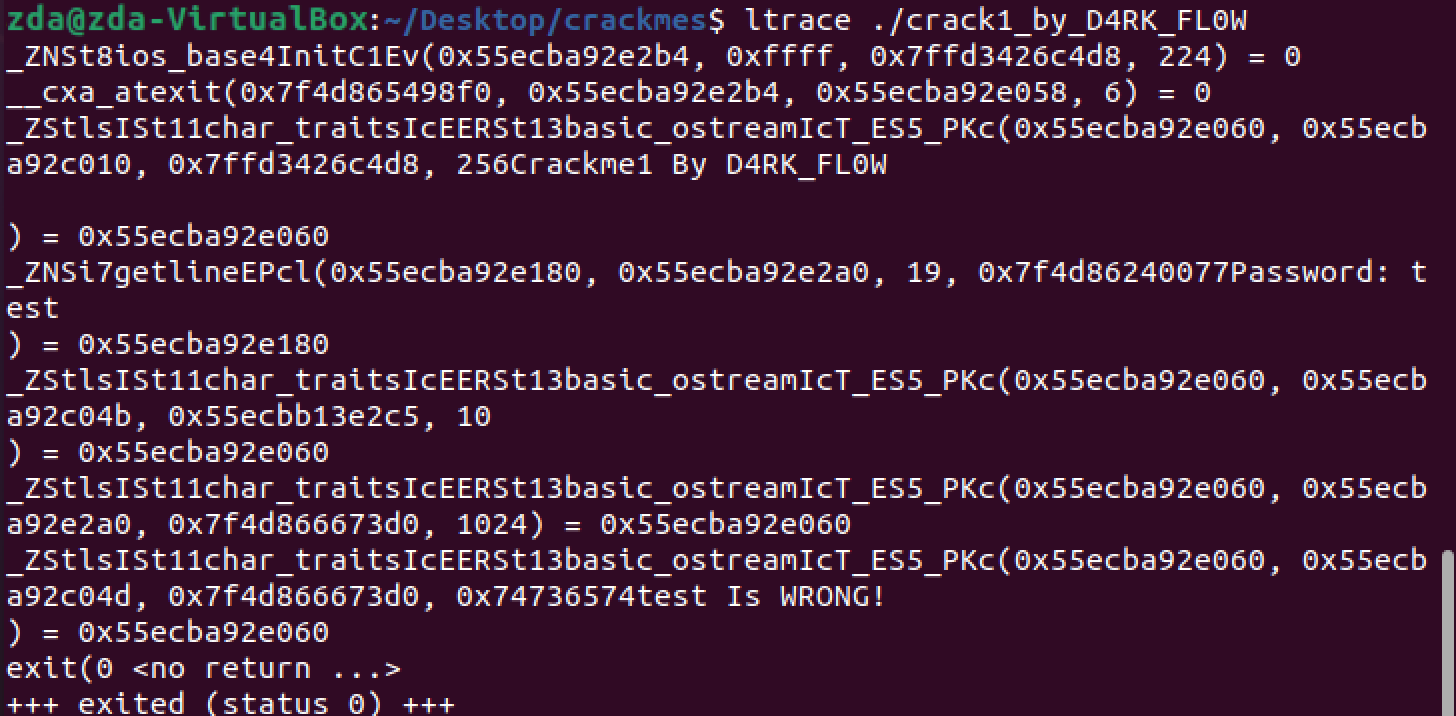
This time we can’t see anything obvious like the “strcmp” call like we saw in Part 1. If you try again using
strace you will get similar results but with a bit more text. Okay, so nothing obvious from
strings,
ltrace, and
strace. We haven’t used a disassembler yet though, so let’s try that. We could use
objdump like we did in Part 1 and that will work just fine, but let’s use
gdb this time to change it up a bit. It is widely used and contains a suite of helpful static and dynamic analysis tools. It also highlights disassembled code in a way that can be helpful visually.
Open up the program with
gdb:
$ gdb crack1_by_D4RK_FL0W
You should now see something like this:
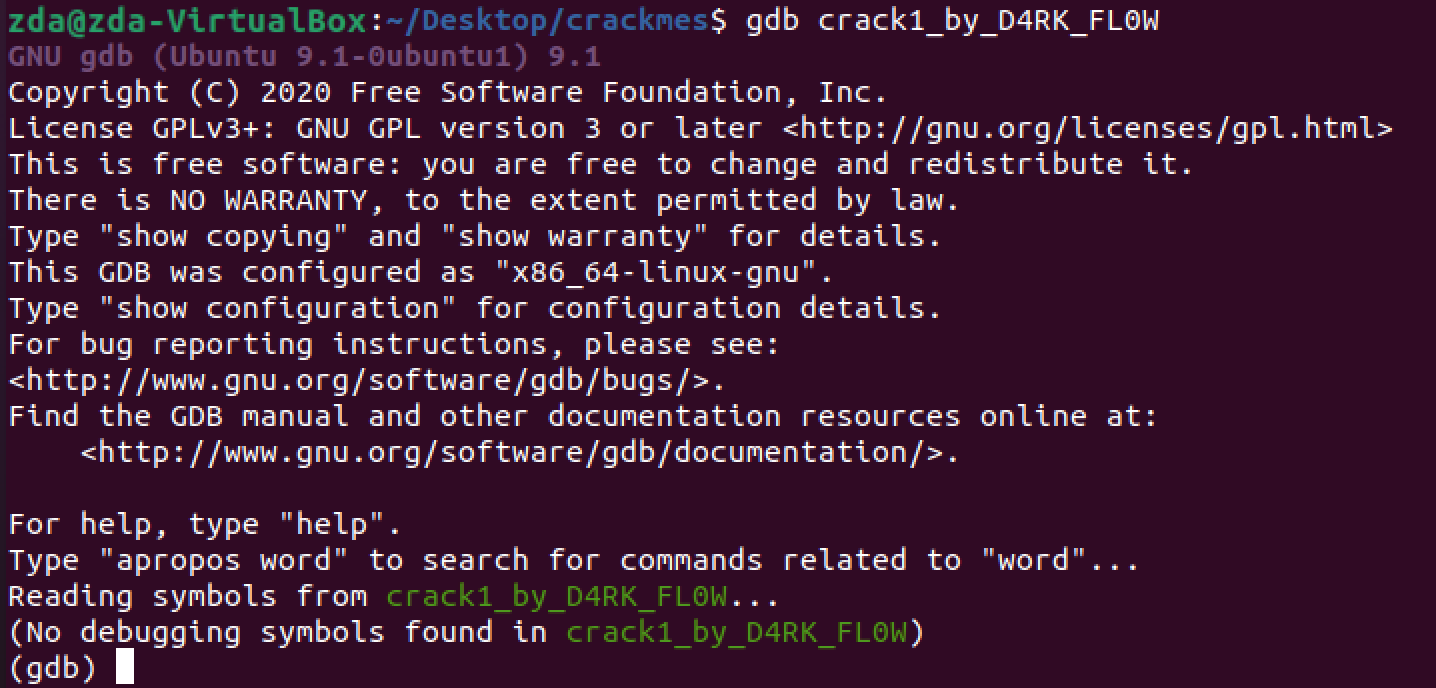
We want to disassemble the code. Since this is a relatively small program and we know it was written in C/C++, let’s target the main function before we do anything else. Type “disass *main” to disassemble the main function:
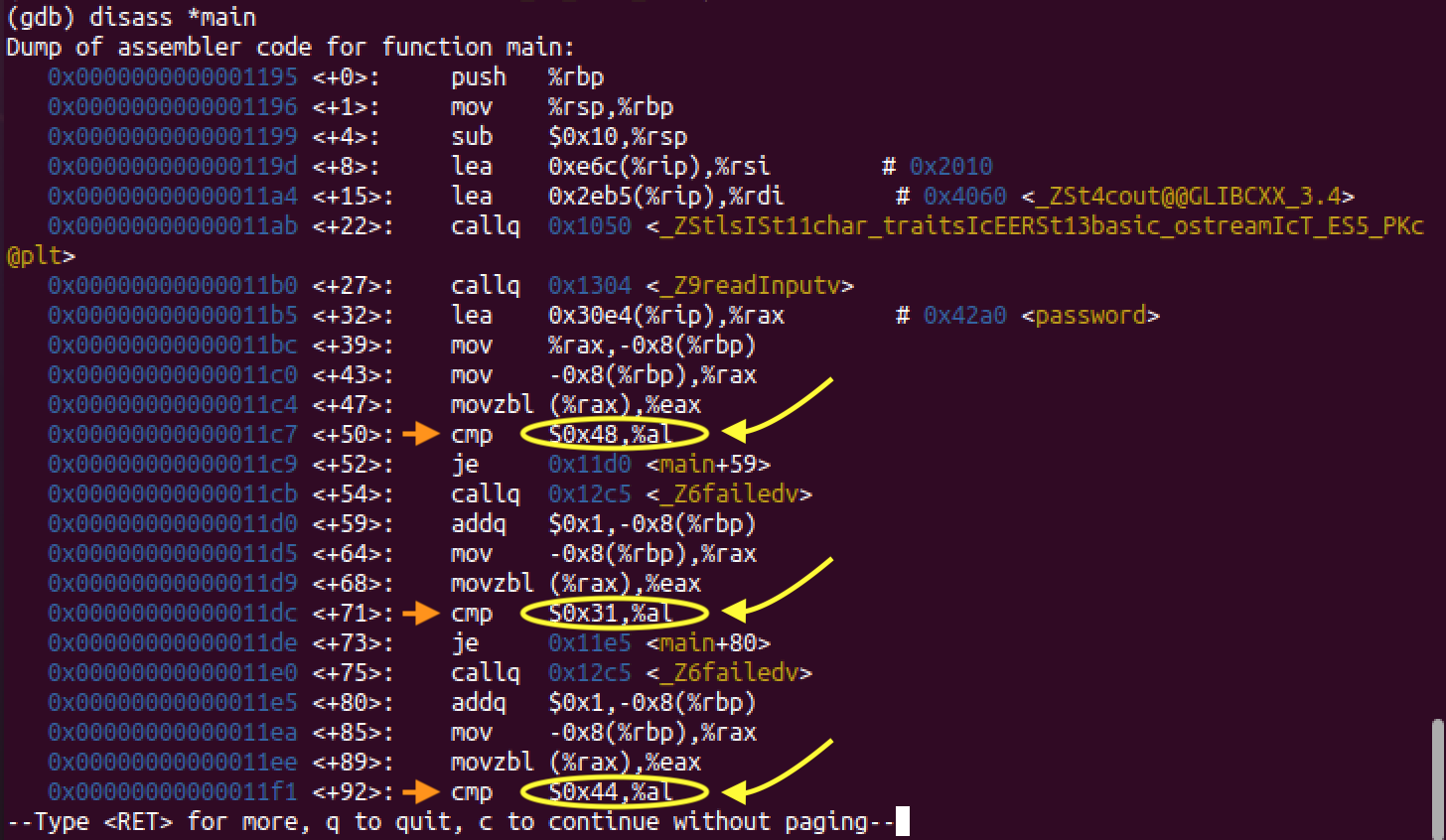
In Part 1 we saw “strcmp” pop up when using
ltrace which tipped us off that the program was comparing our “test” password with another string that we correctly identified as the password “easycrack”. This time we are looking at assembly so there is no “strcmp”, but you can see that there are several lines with “cmp” instructions followed by “je” instructions like this first one:

Read the “cmp” command as “compare”. We use it to compare two values, in this case we are comparing 0x48 with al. Read the “je” command as “jump if equal”. In this case if 0x48 and al are equal to each other, then the program will jump to address 0x11d0 in memory. You can see the memory addresses for each line on the far left.
If the two values are not equal and it doesn’t skip ahead to a new address, the program will execute a function call named “failed”:

This pattern is repeated over and over in the main function, if you hit return, you’ll see even more examples:
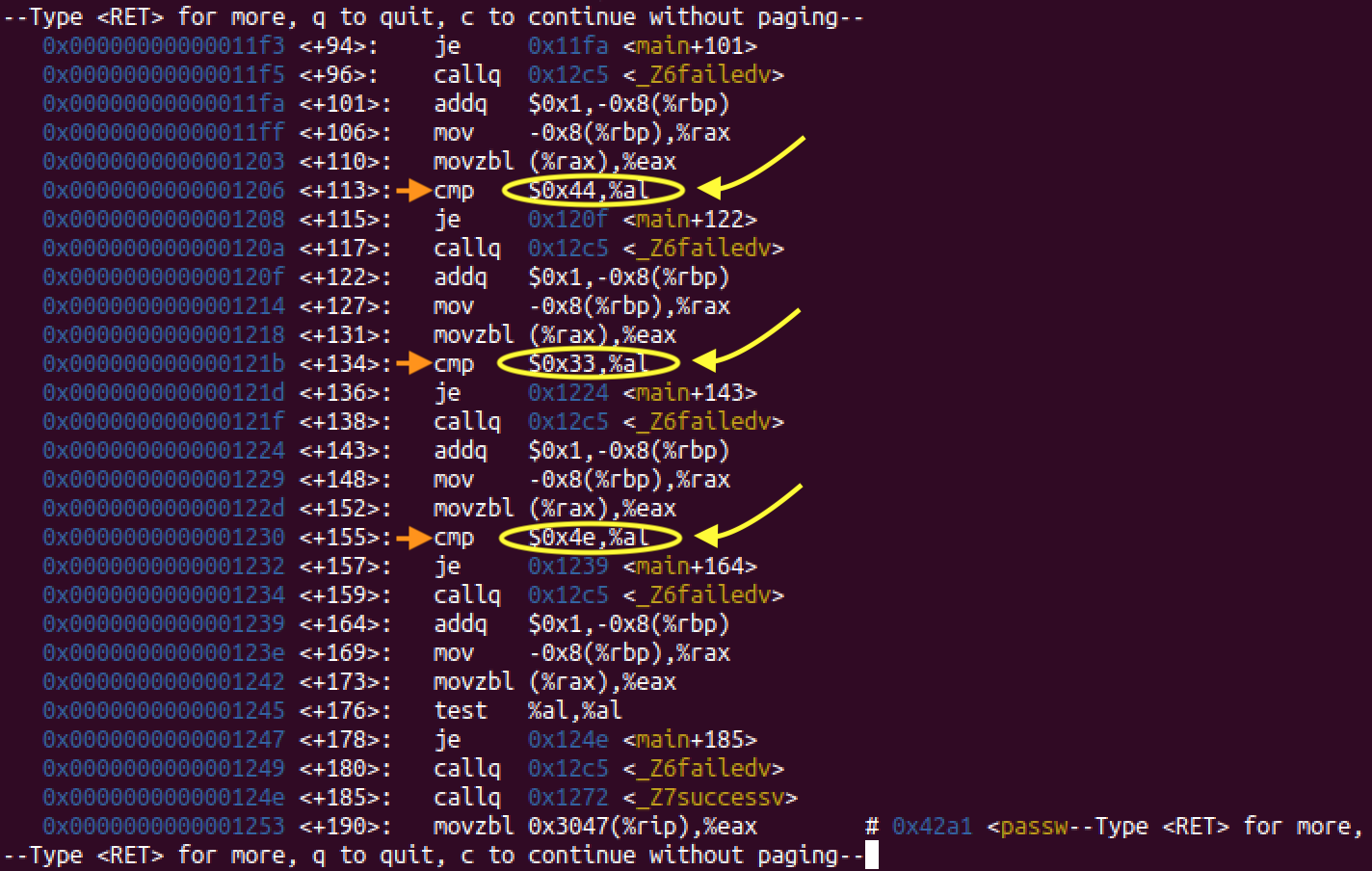
Hitting return once more and we can see that the main function terminates:
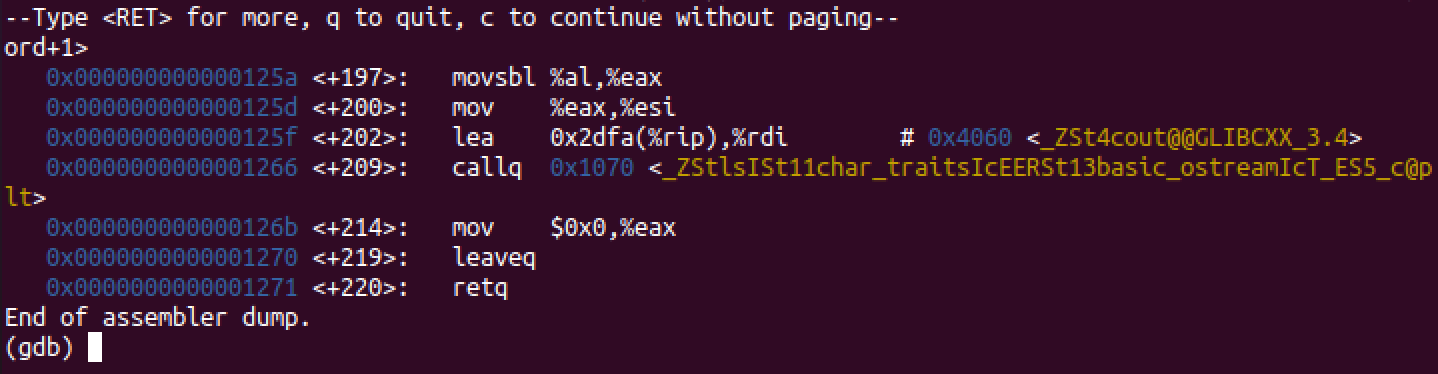
This seems like a candidate for this program checking each character in a given password to the real password. From top to bottom, let’s make note of the hex values from each of these comparisons. If we write them in order (dropping th 0x prefix) we get:
48 31 44 44 33 4e
Could this be related to our password? These are hex values that could potentially represent ascii characters. I like to use this
online hex to ascii converter. Let's convert them to ascii and see what we get:
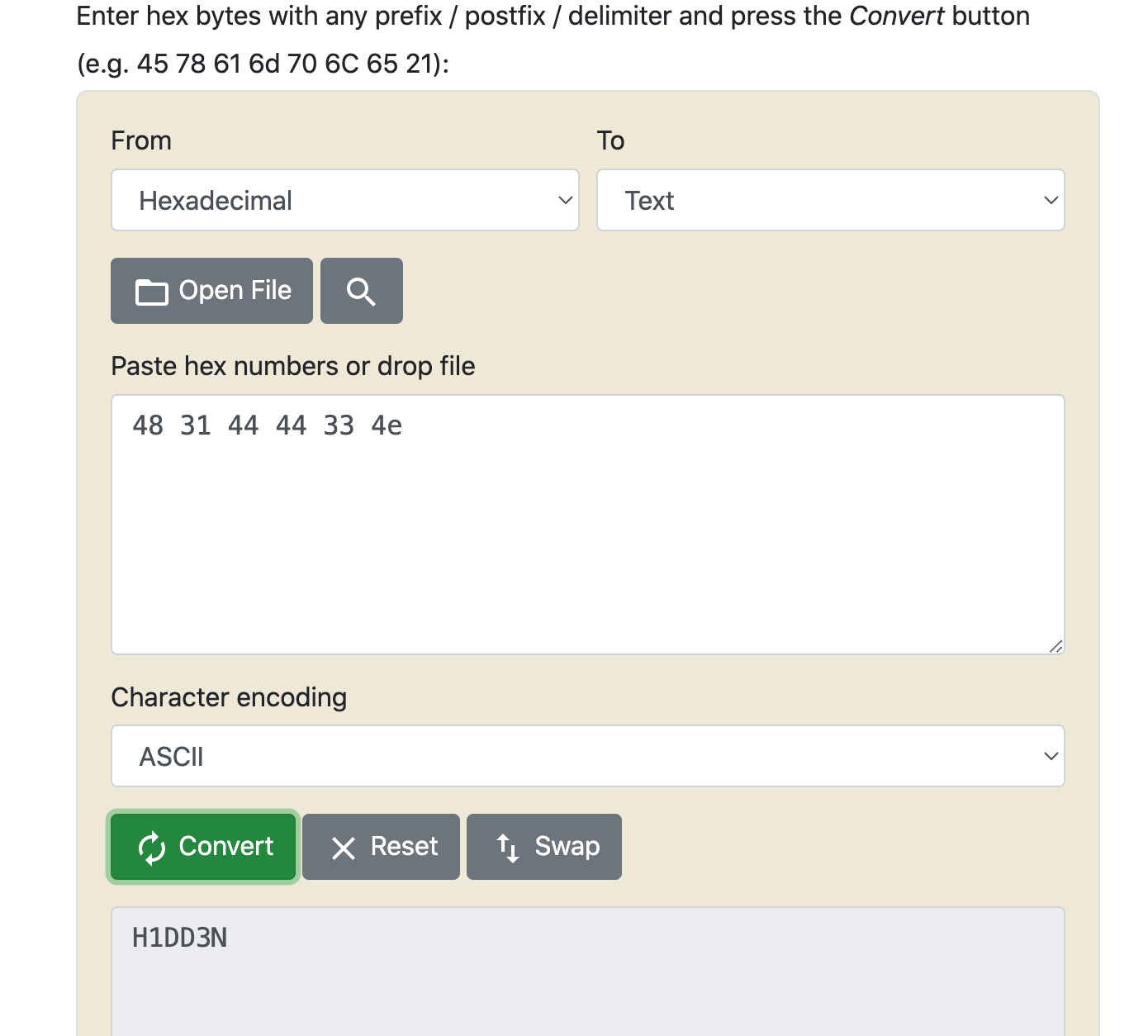
Upon clicking “Convert” we can see the string “H1DD3N”. Considering we are looking for a hidden password and the way D4RKFL0W writes his name, I’d say we’ve found a likely candidate for the password for this crackme. Exit
gdb by typing “quit” and pressing return, and run the program again. Try “H1DD3N” as the password:

We’re on a roll! Two crackmes down (albeit easy ones) without even opening
Ida or
Ghidra.
From here, I’d suggest trying a few more difficulty 1.0 crackmes from
crackmes.one on your own. Don’t be discouraged if you can’t figure them out right away. If one seems too hard, try another and build some confidence. Not all the crackmes labeled with difficulty 1.0 are going to be as straightforward as the ones we tried here. It also wouldn’t hurt to brush up on Intel assembly and C/C++ if you feel you are lacking in this regard. Again, you don’t need to necessarily master these by any means, but you should know the basics.